18.2.2 Cryptographic agility
To cope with possible future advances in cryptology, good security systems are built in a way that makes it easy to replace individual cryptographic algorithms with new ones if needed. This design pattern is called algorithm agility or cryptographic agility (or crypto-agility, for short) and allows maintaining the security of a system, even if a weakness is found in one of its cryptographic primitives.
Bryan Sullivan’s talk on cryptographic agility at the BlackHat security conference [169] nicely illustrates how this concept is implemented in practice, in .NET and Java Cryptography architecture frameworks.
Figure 18.1 shows the .NET framework class SHA512CSP implementing the SHA-512 hash function. The SHA512CSP class inherits from the abstract class SHA512, which, in turn, inherits from the abstract class HashAlgorithm.
The HashAlgorithm base class defines a public method called ComputeHash. This method calls an abstract protected method, HashCore, which does the actual hash computation. Hence, the SHA512SCP class that is derived from the HashAlgorithm class must implement its own HashCore method.
In addition, the HashAlgorithm class implements a class method called Create, which acts as a factory class for classes that derive from HashAlgorithm. The method takes the name of the algorithm to be instantiated and creates a new instance of HashAlgorithm with the desired hash function. A call to the ComputeHash method of the newly created instance performs the actual computation:
HashAlgorithm myHash = HashAlgorithm.Create(desiredAlgorithmName);
byte[] result = myHash.ComputeHash(data);
The following diagram shows the interdependencies between the various classes related to the base class HashAlgorithm:
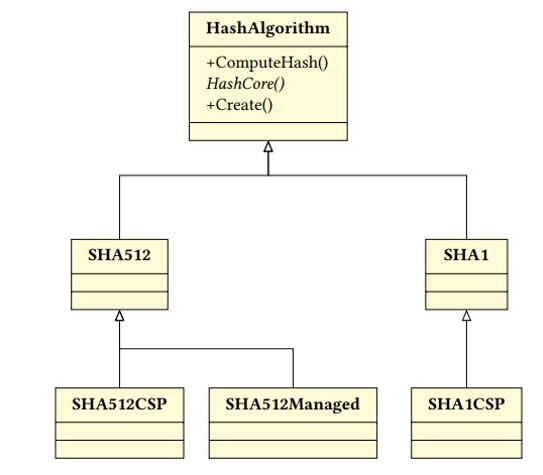
Figure 18.1: Inheritance structure in .NET framework’s cryptography part
The software architecture shown in Figure 18.1 allows developers to write application code without hardcoding the hash function to be used.
Instead, the code can load the name of the desired hash algorithm from a database or a configuration file specified by the system administrator. The administrator, in turn, is now able to select the algorithm and the implementation they want to use. For example, they can easily replace the insecure SHA-1 algorithm with the secure SHA-512 algorithm.
18.2.3 Standby ciphers
During the AES contest, 15 submitted algorithms were accepted and their security was analyzed at 3 public workshops and many more peer-reviewed scientific conferences over the course of 4 years.
Even if we assume that other ciphers offer a security level similar to that of AES, every additional cipher that a TLS endpoint must support increases the development, validation, and maintenance cost. So, why have a second encryption algorithm?
The reason for having a second, standby algorithm is long-term security. If future advances in cryptanalysis uncover a cryptographic weakness in AES, the second algorithm will be readily available as a drop-in replacement for AES.
The TLS protocol accommodates cryptographic agility by allowing Alice and Bob to negotiate the algorithms they want to use in a particular TLS session. The ability to negotiate algorithms, for one thing, helps to achieve compatibility among heterogeneous devices from different vendors: even if Alice and Bob support different sets of algorithms, they can dynamically select a cipher suite they both support as long as their sets overlap. Simultaneously, the ability to negotiate makes it architecturally easy to include a standby cipher that Alice and Bob can immediately switch to if the mandatory-to-implement cipher becomes cryptographically weak.
In addition – as David McGrew, Anthony Grieco, and Yaron Sheffer outline in [113] – allowing to negotiate algorithms (in other words, providing cryptographic agility) counteracts the proliferation of cryptographic protocols.
Typically, an abundance of cryptographic protocols is worse than an abundance of cryptographic algorithms because it is much easier to replace a cryptographic algorithm than a cryptographic protocol. Why? Because the complexity of a cryptographic algorithm is isolated by a simple interface, while a protocol’s complexity is not isolated at all – a protocol must interact with the network stack layers below and above.
The standby cipher must meet the same security and efficiency standards as the mandatory-to-implement cipher. In particular, the standby cipher must undergo an extensive public review process where the cryptographic community has the opportunity to carefully investigate the cipher for any potential security weaknesses. Moreover, the standby cipher must fulfill two additional requirements:
- Its design, that is, its mathematical working principles, must be as independent as possible from that of the mandatory-to-implement cipher. This way, the cryptanalytic advances that weaken the mandatory-to-implement cipher will have the least effect on the standby cipher.
- The mandatory-to-implement cipher is optimized for the existing hardware. The standby cipher must be efficiently computable on the existing hardware as well.
We will now take a look at the standby cipher for the AES.
Leave a Reply